社区精选|在 Vue 中控制表单输入
用户在输入框中输入,text 会发生变化。 text 发生变化,输入框的值也随之变化。
const text = ref():作为 v-model 可响应式的值。 v-model="text":将 v-model 添加到分配有 text 的输入表单标签中。
<script setup>
import { ref } from 'vue'
const text = ref('Unknown') // Step 1: create data bus
</script>
<template>
<!-- Step 2: assign data bus to v-model -->
<input v-model="text" type="input" />
<div>{{ text }}</div>
</template>

<input v-bind:value="text" type="text" />
<input :value="text" type="text" />
<script setup>
import { ref } from 'vue'
const text = ref('Unknown')
</script>
<template>
<input :value="text" type="input" />
<div>{{ text }}</div>
</template>
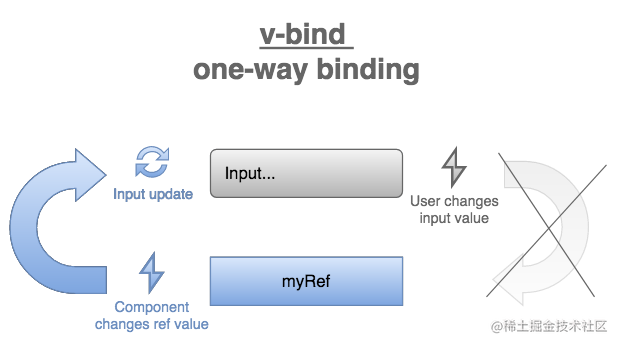
<input v-model="text" type="text" />
<input :value="text" type="text" />
<script setup>
import { ref } from 'vue'
const text = ref('Unknown')
</script>
<template>
<input
:value="text"
@input="text = $event.target.value"
type="input"
/>
<div>{{ text }}</div>
</template>
<script setup>
import { reactive } from 'vue'
const person = reactive({ firstName: 'John', lastName: 'Smith' })
</script>
<template>
<input v-model="person.firstName" type="input" />
<input v-model="person.lastName" type="input" />
<div>Full name is {{ person.firstName }} {{ person.lastName }}</div>
</template>
<script setup>
import { ref } from 'vue'
const longText = ref("Well... here's my story. One morning...")
</script>
<template>
<textarea v-model="longText" />
<div>{{ longText }}</div>
</template>
<script setup>
import { ref } from 'vue'
const employeeId = ref('2')
</script>
<template>
<select v-model="employeeId">
<option value="1">Jane Doe</option>
<option value="2">John Doe</option>
<option value="3">John Smith</option>
</select>
<div>Selected id: {{ employeeId }}</div>
</template>
<script setup>
import { ref } from 'vue'
const employee = ref('Jane Doe')
</script>
<template>
<select v-model="employee">
<option>Jane Doe</option>
<option>John Doe</option>
<option>John Smith</option>
</select>
<div>Selected: {{ employee }}</div>
</template>
<input ref="checked" type="checkbox" />
<script setup>
import { ref } from 'vue'
const checked = ref(true)
</script>
<template>
<label><input v-model="checked" type="checkbox" />Want a pizza?</label>
<div>{{ checked }}</div>
</template>
<input
v-model="checked"
true-value="Yes!"
false-value="No"
/>
<script setup>
import { ref } from 'vue'
const answer = ref('Yes!')
</script>
<template>
<label>
<input v-model="answer" type="checkbox" true-value="Yes!" false-value="No" />
Want a pizza?
</label>
<div>{{ answer }}</div>
</template>
<input type="radio" v-model="option" value="a" />
<input type="radio" v-model="option" value="b" />
<input type="radio" v-model="option" value="c" />
<script setup>
import { ref } from "vue"
const color = ref("white")
</script>
<template>
<label><input type="radio" v-model="color" value="white" />White</label>
<label><input type="radio" v-model="color" value="red" />Red</label>
<label><input type="radio" v-model="color" value="blue" />Blue</label>
<div>T-shirt color: {{ color }}</div>
</template>
<script setup>
import { ref } from "vue"
const color = ref("white")
const COLORS = [
{ option: "white", label: "White" },
{ option: "black", label: "Black" },
{ option: "blue", label: "Blue" },
]
</script>
<template>
<label v-for="{ option, label } in COLORS" :key="option">
<input type="radio" v-model="color" :value="option" /> {{ label }}
</label>
<div>T-shirt color: {{ color }}</div>
</template>
v-model 修饰符
除了在绑定表单输入方面做得很好之外,v-model 还有一个额外的功能,叫做修饰符。
修饰符是应用于 v-model 的一段逻辑,用于自定义其行为。修饰符通过使用点语法 v-model.<modifier>应用于 v-model,例如 v-mode.trim。
默认情况下,Vue 提供了 3 个修饰符,trim、number 和 lazy。
trim
清除一个字符串是指删除字符串开头和结尾的空白处。例如,清除应用于' Wow! '的结果是'Wow!'。
<script setup>
import { ref } from 'vue'
const text = ref('')
</script>
<template>
<input v-model.trim="text" type="text" />
<pre>"{{ text }}"</pre>
</template>
number
v-model.number 修饰符在输入表单的值上应用一个数字解析器。
<script setup>
import { ref } from "vue";
const number = ref("");
</script>
<template>
<input v-model.number="number" type="text" />
<div>{{ typeof number }}</div>
</template>
但是如果你在 input 中引入一个非数值,比如'abc',那么 number 就会被分配为相同的值'abc'。
lazy
默认情况下,当绑定的值更新时,v-model 会使用 input 事件。但如果使用修饰符 v-model.lazy,你可以将该事件改为 change 事件。
input 是每当你在输入表单键入时就会触发。 change 是只有当你把焦点从输入表单移开时,才会触发。在输入表单里输入并不会触发 change 事件。
<script setup>
import { ref } from 'vue'
const text = ref('Unknown')
</script>
<template>
<input v-model.lazy="text" type="input" />
<div>{{ text }}</div>
</template>
如果你有一个许多输入字段和大量状态的表单,你可以应用 lazy 修饰符来禁用用户输入时的实时响应。这可以防止输入时页面卡住。
总结
v-model 将表单输入与 ref 或响应式对象进行绑定。
绑定是通过两个简单的步骤实现的:
首先,通过 const text = ref('')创建 ref。 其次,将 ref 分配给 v-model 属性:<input v-model="text" type="text" />。
以上就是本文的全部内容,如果对你有所帮助,欢迎点赞、收藏、转发~
点击左下角阅读原文,到 SegmentFault 思否社区 和文章作者展开更多互动和交流,“公众号后台“回复“ 入群 ”即可加入我们的技术交流群,收获更多的技术文章~
- END -


关注公众号:拾黑(shiheibook)了解更多
赞助链接:
关注数据与安全,洞悉企业级服务市场:https://www.ijiandao.com/
四季很好,只要有你,文娱排行榜:https://www.yaopaiming.com/
让资讯触达的更精准有趣:https://www.0xu.cn/
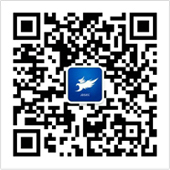
随时掌握互联网精彩